- TThread a line of execution within a program. Each program can have multiple associated threads. Each thread has a priority which is used by the thread scheduler to determine which thread must run first. Java provides a thread class that has various method calls in order to manage the behavior of threads by providing constructors and methods to perform operations on threads.
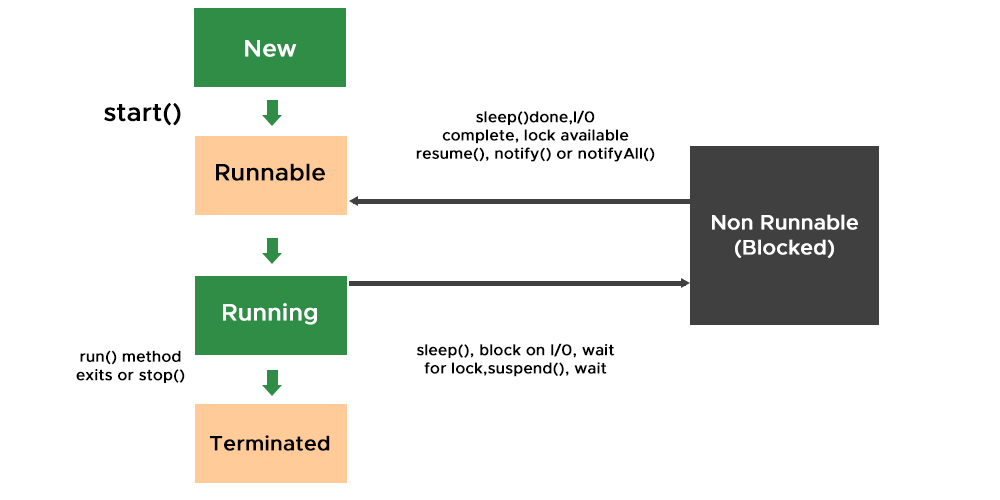
Ways of creating threads
- Creating own class which is extending to parent Thread class
- Implementing the Runnable interface.
class MyThread extends Thread {
// Method 1
// Run() method for our thread
public void run()
{
// Print statement
System.out.println(
"Thread is running created by extending
to parent Thread class");
}
// Method 2
// Main driver method
public static void main(String[] args)
{
// Creating object of our thread class
inside main()
// method
MyThread myThread = new MyThread();
// Starting the thread
myThread.start();
}
}
output :
Thread is running created by
extending to
parent Thread class
- Illustration 2:
class ThreadUsingInterface
implements Runnable {
// Method 1
// run() method for the thread
public void run()
{
// Print statement
System.out.println("Thread is created
using Runnable interface");
}
// Method 2
// Main driver method
public static void main(String[] args)
{
// Creating object of our thread class
inside main()
// method
ThreadUsingInterface obj
= new ThreadUsingInterface();
// Passing the object to thread in main()
Thread myThread = new Thread(obj);
// Starting the thread
myThread.start();
}
}
output :
Thread is created
using Runnable interface
Thread Class in Java
- A thread is a program that starts with a method() frequently used in this class only known as the start() method. This method looks out for the run() method which is also a method of this class and begins executing the body of the run() method. Here, keep an eye over the sleep() method which will be discussed later below..
- Note: Every class that is used as thread must implement Runnable interface and over ride it’s run method.
Syntax:
public class Thread extends Object
implements Runnable
Constructors of this class are as follows:
Constructor | Action Performed |
---|---|
Thread() | Allocates a new Thread object. |
Thread(Runnable target) | Allocates a new Thread object. |
Thread(Runnable target, String name) | Allocates a new Thread object. |
Thread(String name) | Allocates a new Thread object. |
Thread(ThreadGroup group, Runnable target) | Allocates a new Thread object. |
Thread(ThreadGroup group, Runnable target, String name) | Allocates a new Thread object so that it has targeted as its run object, has the specified name as its name, and belongs to the thread group referred to by a group. |
Thread(ThreadGroup group, Runnable target, String name, long stackSize) | Allocates a new Thread object so that it has targeted as its run object, has the specified name as its name, and belongs to the thread group referred to by group, and has the specified stack size. |
Thread(ThreadGroup group, String name) | Allocates a new Thread object. |
Example:
// Java program Demonstrating Methods of
Thread class
// Importing package
package generic;
class Helper implements Runnable {
//
public void run() {
// Try block to check for exceptions
try {
// Print statement
System.out.println
("thread2 going to sleep for 5000");
// Making thread sleep for 0.5 seconds
Thread.sleep(5000);
}
// Catch block to handle exception
catch (InterruptedException e) {
// Print statement
System.out.println("Thread2 interrupted");
}
}
}
public class Test implements Runnable {
// Method 1
// run() method of this class
public void run() {
// Thread run() method
}
// Method 2
// Main driver method
public static void main(String[] args) {
// Making objects of class 1 and 2 in
main() method
Test obj = new Test();
Helper obj2 = new Helper();
// Creating 2 threads in main() method
Thread thread1 = new Thread(obj);
Thread thread2 = new Thread(obj2);
// Moving thread to runnable states
thread1.start();
thread2.start();
// Loading thread 1 in class 1
ClassLoader loader = thread1
.getContextClassLoader();
// Creating 3rd thread in main() method
Thread thread3 = new Thread(new Helper());
// Getting number of active threads
System.out.println(Thread.activeCount());
thread1.checkAccess();
// Fetching an instance of this thread
Thread t = Thread.currentThread();
// Print and display commands
System.out.println(t.getName());
System.out.println("Thread1 name:
" + thread1.getName());
System.out.println("Thread1 ID:
" + thread1.getId());
System.out.println("Priority of thread1 =
" + thread1.getPriority());
// Getting the state of thread 1 using
getState() method
// and printing the same
System.out.println(thread1.getState());
thread2 = new Thread(obj2);
thread2.start();
thread2.interrupt();
System.out.println("Is thread2 interrupted?
" + thread.interrupted() );
System.out.println("Is thread2 alive?
" + thread2.isAlive());
thread1 = new Thread(obj);
thread1.setDaemon(true);
System.out.println
("Is thread1 a daemon thread?
" + thread1.isDaemon());
System.out.println("Is thread1 interrupted?
" + thread1.isInterrupted());
System.out.println("thread1 waiting for
thread2 to join");
try {
thread2.join();
}
catch (InterruptedException e) {
// Display the exception along with
line number
// using printStackTrace() method
e.printStackTrace();
}
// Now setting the name of thread1
thread1.setName("child thread xyz");
// Print and display command
System.out.println("New name set for thread 1"
+ thread1.getName());
// Setting the priority of thread1
thread1.setPriority(5);
thread2.yield();
System.out.println(thread1.toString());
// Getting list of active thread in
current thread's group
Thread[] tarray = new Thread[3];
Thread.enumerate(tarray);
// Display commands
System.out.println
("List of active threads:");
System.out.printf("[");
// Looking out using for each loop
for (Thread thread : tarray) {
System.out.println(thread);
}
// Display commands
System.out.printf("]\n");
System.out.println
(Thread.getAllStackTraces());
ClassLoader classLoader = thread1
.getContextClassLoader();
System.out.println
(classLoader.toString());
System.out.println(thread1
.getDefaultUncaughtExceptionHandler());
thread2.setUncaughtExceptionHandler
(thread1.getDefaultUncaughtExc
eptionHandler());
thread1.
setContextClassLoader
(thread2.getContextClassLoader());
thread1.setDefaultUncaughtExceptionHandle
(thread2.getUncaughtExceptionHandler());
thread1 = new Thread(obj);
StackTraceElement[] trace = thread1
.getStackTrace();
System.out.println("Printing stack
trace elements for thread1:");
for (StackTraceElement e : trace) {
System.out.println(e);
}
ThreadGroup grp
= thread1.getThreadGroup();
System.out.println("ThreadGroup to which
thread1 belongs " + grp.toString());
System.out.println(thread1
.getUncaughtExceptionHandler());
System.out.println
("Does thread1 holds Lock? "
+ thread1.holdsLock(obj2));
Thread.dumpStack();
}
}
Output:
3
main
Thread1 name: Thread-0
Thread1 ID: 10
Priority of thread1 = 5
RUNNABLE
Is thread2 interrupted? false
Is thread2 alive? true
Is thread1 a daemon thread? true
Is thread1 interrupted? false
thread1 waiting for thread2 to join
thread2 going to sleep for 5000 ms
thread2 going to sleep for 5000 ms
Thread2 interrupted
New name set for thread 1child thread xyz
Thread[child thread xyz, 5, main]
List of active threads:
[Thread[main, 5, main]
Thread[Thread-1, 5, main]
null
]
{Thread[Signal Dispatcher, 9, system]=
[Ljava.lang.StackTraceElement;@33909752,
Thread[Thread-1, 5, main]
=[Ljava.lang.StackTraceElement;@55f96302,
Thread[main, 5, main]
=[Ljava.lang.StackTraceElement;@3d4eac69,
Thread[Attach Listener, 5, system]
=[Ljava.lang.StackTraceElement;@42a57993,
Thread[Finalizer, 8, system]
=[Ljava.lang.StackTraceElement;@75b84c92,
Thread[Reference Handler, 10, system]
=[Ljava.lang.StackTraceElement;@6bc7c054}
sun.misc.Launcher$AppClassLoader@73d16e93
null
Printing stack trace elements for thread1:
ThreadGroup to which thread1
belongs java.lang
.ThreadGroup[name=main, maxpri=10]
java.lang.ThreadGroup[name=main, maxpri=10]
Does thread1 holds Lock? false
java.lang.Exception: Stack trace
at java.lang.Thread.dumpStack(Unknown Source)
at generic.Test.main(Test.java:111)